An Extension function provides the ability to extend a class with new functionality without having to inherit from the class or use any type of design pattern such as Decorator.
Extension function is one of my favorite features in Kotlin language and I have been using it for a long time. In this post, I will list down all the extension functions that I found useful and have been using it in my Android projects.
Button Extensions
/**
* Button enabling/disabling modifiers
*/
fun Button.disableButton() {
isEnabled = false
alpha = 0.7f
}
fun Button.enableButton() {
isEnabled = true
alpha = 1.0f
}
// Usage
loginButton.disableButton()
loginButton.enableButton()
View Extensions
/**
* Visibility modifiers and check functions
*/
fun View.isVisibile(): Boolean = visibility == View.VISIBLE
fun View.isGone(): Boolean = visibility == View.GONE
fun View.isInvisible(): Boolean = visibility == View.INVISIBLE
fun View.makeVisible() {
visibility = View.VISIBLE
}
fun View.makeGone() {
visibility = View.GONE
}
fun View.makeInvisible() {
visibility = View.INVISIBLE
}
/**
* Hides the soft input keyboard from the screen
*/
fun View.hideKeyboard(context: Context?) {
val inputMethodManager = context?.getSystemService(Context.INPUT_METHOD_SERVICE) as InputMethodManager
inputMethodManager.hideSoftInputFromWindow(this.windowToken, 0)
}
// Usage
submitButton.isVisible() // Returns true if the Button is visible
submitButton.isGone() // Returns true if the Button is gone
submitButton.isInvisible() // Returns true if the Button is invisible
submitButton.makeVisible() // Sets the button's visibility to visible
submitButton.makeGone() // Sets the button's visibility to gone
submitButton.makeInvisible() // Sets the button's visibility to invisible
// Hides the keyboard on Button click
submitButton.setOnClickListener {
it.hideKeyboard(context)
}
ImageView Extensions
/**
* Loads URL into an ImageView using Picasso
*
* @param url URL to be loaded
*/
fun ImageView.loadFromUrl(url: String) {
Picasso.get().load(url).into(this)
}
/**
* Loads URL into an ImageView using Glide
*
* @param url URL to be loaded
*/
fun ImageView.loadFromUrl(url: String, context: Context) {
Glide.with(context).load(url).into(this)
}
// Usage
// Picasso
userProfileView.loadFromUrl("https://goo.gl/images/C7X8nb")
// Glide
userProfileView.loadFromUrl("https://goo.gl/images/C7X8nb", context)
Activity Extensions
/**
* Check if the Internet connectivity is available
*/
fun Context.isInternetAvailable(): Boolean {
val connectivityManager = getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager
val activeNetworkInfo = connectivityManager.activeNetworkInfo
return activeNetworkInfo != null && activeNetworkInfo.isConnected
}
// Usage
if (isInternetAvailable()) {
// Do stuff here
}
Snackbar Extensions
/**
* Shows the Snackbar inside an Activity or Fragment
*
* @param messageRes Text to be shown inside the Snackbar
* @param length Duration of the Snackbar
* @param f Action of the Snackbar
*/
fun View.showSnackbar(@StringRes messageRes: Int, length: Int = Snackbar.LENGTH_LONG, f: Snackbar.() -> Unit) {
val snackBar = Snackbar.make(this, resources.getString(messageRes), length)
snackBar.f()
snackBar.show()
}
/**
* Adds action to the Snackbar
*
* @param actionRes Action text to be shown inside the Snackbar
* @param color Color of the action text
* @param listener Onclick listener for the action
*/
fun Snackbar.action(@StringRes actionRes: Int, color: Int? = null, listener: (View) -> Unit) {
setAction(actionRes, listener)
color?.let { setActionTextColor(color) }
}
// Usage
// Shows the Snackbar having text and long duration
constraintLayout.showSnackbar(R.string.download_complete) {}
// Shows the Snackbar having text and short duration
constraintLayout.showSnackbar(R.string.download_complete, Snackbar.LENGTH_SHORT) {}
// Shows the Snackbar with text and action
constraintLayout.showSnackbar(R.string.download_complete) {
action(R.string.open) { Log.e("TAG", "Action item clicked") }
}
EditText Extensions
/**
* Adds TextWatcher to the EditText
*/
fun EditText.onTextChanged(listener: (String) -> Unit) {
this.addTextChangedListener(object : TextWatcher {
override fun afterTextChanged(s: Editable?) {
listener(s.toString())
}
override fun beforeTextChanged(s: CharSequence?, start: Int, count: Int, after: Int) {}
override fun onTextChanged(s: CharSequence?, start: Int, before: Int, count: Int) {}
})
}
// Usage
editTextName.onTextChanged { s ->
Log.e("TAG", "Name length: ${s.length}")
}
Material Design Component Chip Extensions
fun Chip.check() {
isChecked = true
}
fun Chip.unCheck() {
isChecked = false
}
// Usage
contactChip.check()
contactChip.unCheck()
How to use Extension functions
It occurred to me that I’ve read a lot of articles about extension functions but nobody wrote about how to actually use it in the project and hence was confused for some time.
If you are on the same page as I was sometimes ago😬, don’t worry. I am writing down how to use Extension functions in your Android project step-by-step. These are the steps that I follow and you are free to follow your own
Step 1
Create a new package named extensions
Step 2
Create a new Kotlin file named ViewExtensions (The name of the file should be related to the extension functions you are going to add so that you can easily locate them when the project grows big. We are going to add extension functions related to Views so we have named it ViewExtensions)
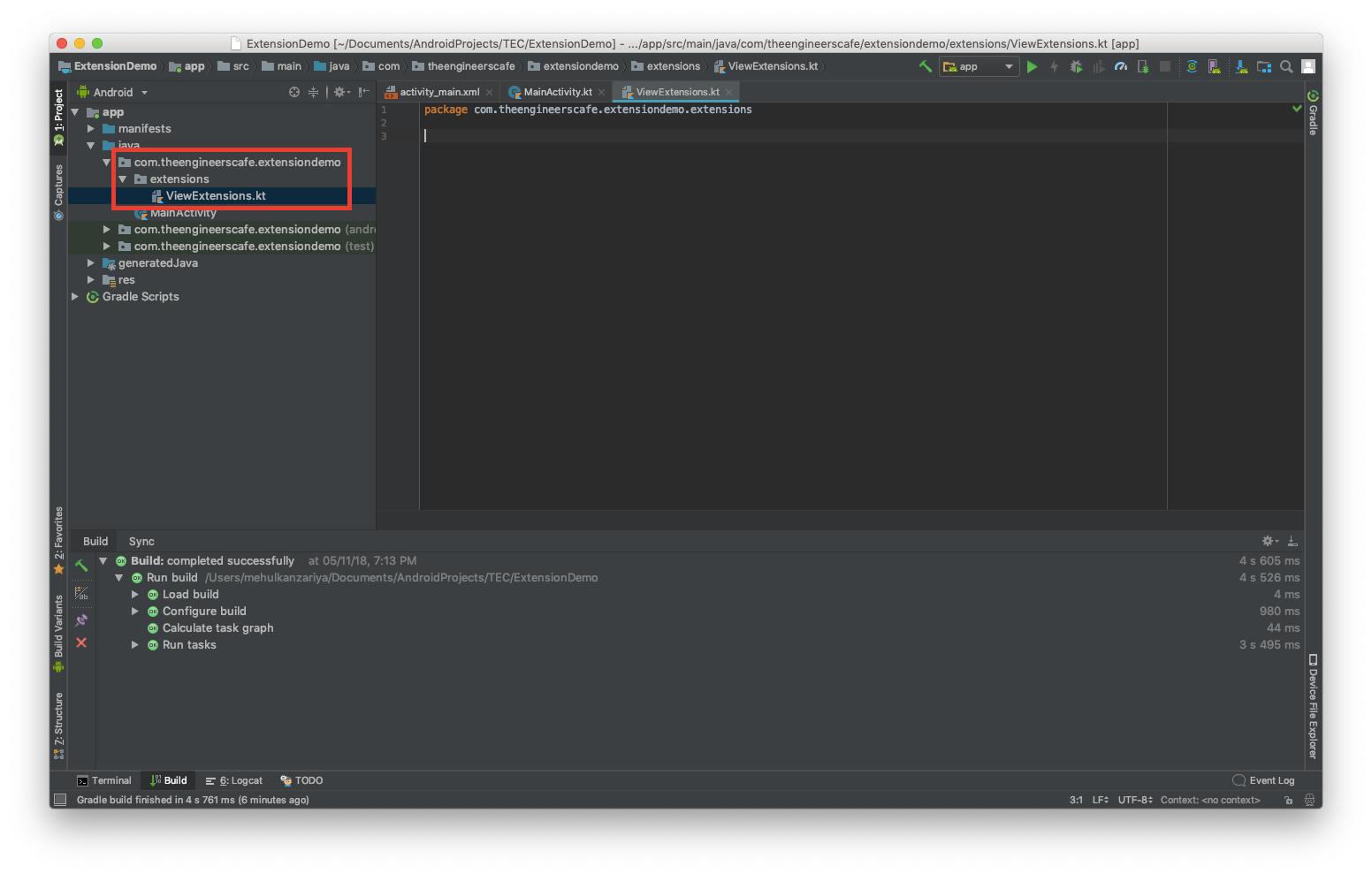
Step 3
Add all the ViewExtensions that we created above to this file.
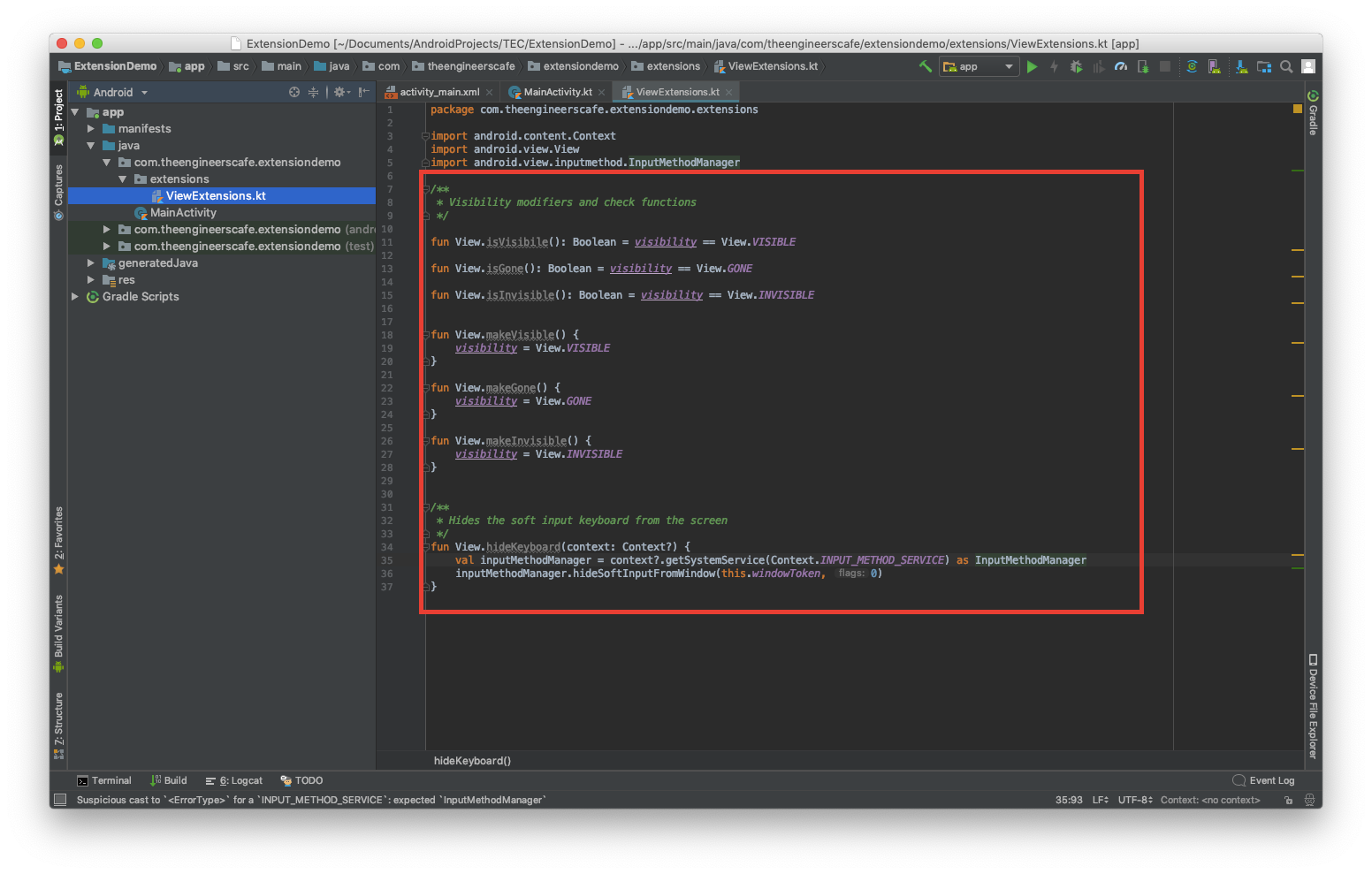
Step 4
Open the Activity or Fragment and use the extension function as shown below.
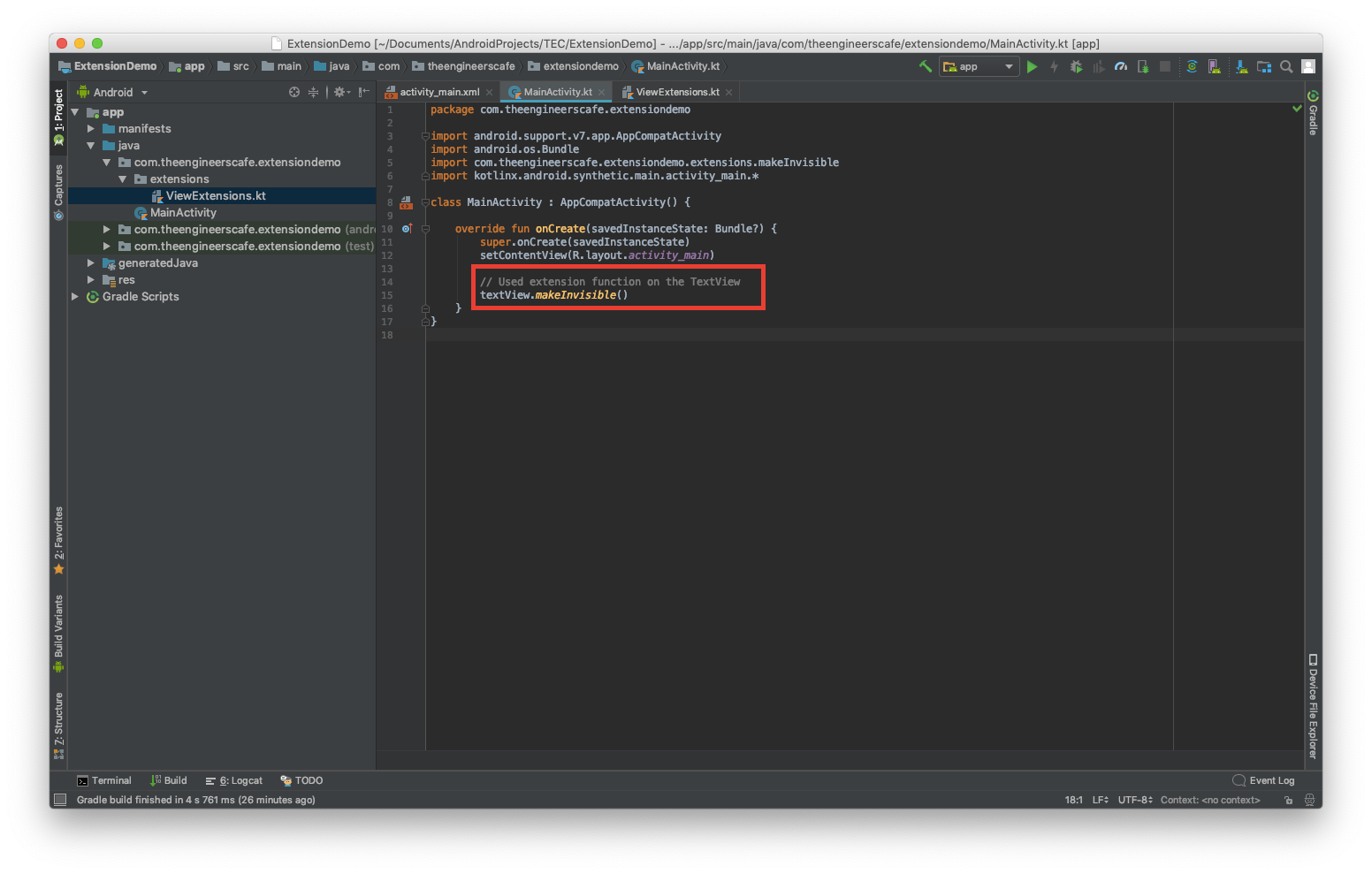
You can follow the same process for different extension functions.
In this post, I have listed down the extension functions that I’ve already used. I will be adding more in the future. Also, if you have some other useful extension functions, write it down in the comments section and I will add it in the post.
If you think the above functions are not implemented properly or can be optimized further, please write down your suggestions in the comments section below.
Happy coding 🙂